Accept payments via API
Use our APIs and build your own payment experience if you want full control over the look and feel of your checkout page.
Building your own payment experience requires significantly more effort for handling all alternative flows, while Payrails provides built-in components that you can customize. So if you'd rather not build your own payment experience, check our Drop-in solution.
With our API-only solution, you have two options for accepting card payments:
- Use our Secure fields card tokenization to collect the card details. This helps you ensure PCI compliance, as you're only required to submit SAQ A.
- Collect and pass raw card data. This requires you to assess your PCI compliance according to SAQ D, the most extensive form of self-certification.
On this page, we describe server-side integration and the client-side implementation that you have to build. Please note this is just an example of what Payrails can offer so that you picture the process better. If you have specific requirements on how you would prefer to accept payments, let us know and we'll guide you throw our API reference.
To get started, make sure you have an API key and are able to Request an access token.
Fetch the payment options & initialize your checkout
To initialize your payment form with Payrails, you need to achieve 3 operations:
- Create a workflow execution. The execution is the equivalent of a customer payment session, so you want to save the
executionId
as a reference to perform the next steps. - Lookup payment options available for the customer in this context. Eventually, you want to bundle this operation with the workflow creation as an
initialAction
to save an API call (like in the example above). - Initialize a client SDK to collect card information on the client side. Eventually, you want to perform this call in parallel to optimize your latency (like in the example above).
Beware that every time the payment data changes (e.g. applying a discount), you want to Lookup payment options again because the payment methods and instruments could be different!
(Optional) Tokenize a new card
This step happens when the customer selects a new card as a payment instrument to authorize with. It is described in detail in the Payrails SDK guide.
Make sure to save the tokenized card output provided by the SDK, you will need it for the next step.
Authorize a payment
When the customer chooses a payment method and an instrument and then clicks the "Pay" button of your checkout form, it is time to Authorize a payment!
This process is asynchronous, after your server-side application sent the authorization request, it will immediately receive an HTTP 202 ACCEPTED
acknowledgment from Payrails.
It doesn't mean that your request was successful, but that it is in progress and Payrails will notify you about the success (or failure) later.
Meanwhile, you can decide to Receive notifications or long poll the execution to fetch the status with the Get an execution by ID endpoint.
For understanding the possible results of payment authorization, you should check the Result Codes page.
(Optional) Handle redirects for 3-D Secure
An authorization with cards might require a 3D Secure challenge to be resolved.
Since the 3-D Secure challenge happens in a WebView (mobile) or a webpage (web), the user journey will leave your domain. Therefore, you wanna make sure your authorization request specifies in the returnInfo
which return URL the user shall be redirected to after the 3-D Secure challenge is resolved.
After the authorization request is placed, you will be informed by a notification or long poll of the execution to fetch the status if a 3DS redirect was requested.
"actionRequired": "3ds",
"links": {
"3ds": "https://api.payrails.io/public/redirect/merchant/..."
}
After fetching the 3DS URL, your client will open a WebView (mobile) or redirect the user to a webpage (web) where the challenge will take place.
When the challenge is resolved, Payrails will take your client back to the address you specified in the returnInfo
. It doesn't mean that your authorization was successful, but that it is in progress and Payrails will notify you about the success (or failure) later (similar to an authorization without 3DS).
(Optional) Handle redirects for Alternative Payment Methods (APMs) with Hosted Payment Pages (HPP)
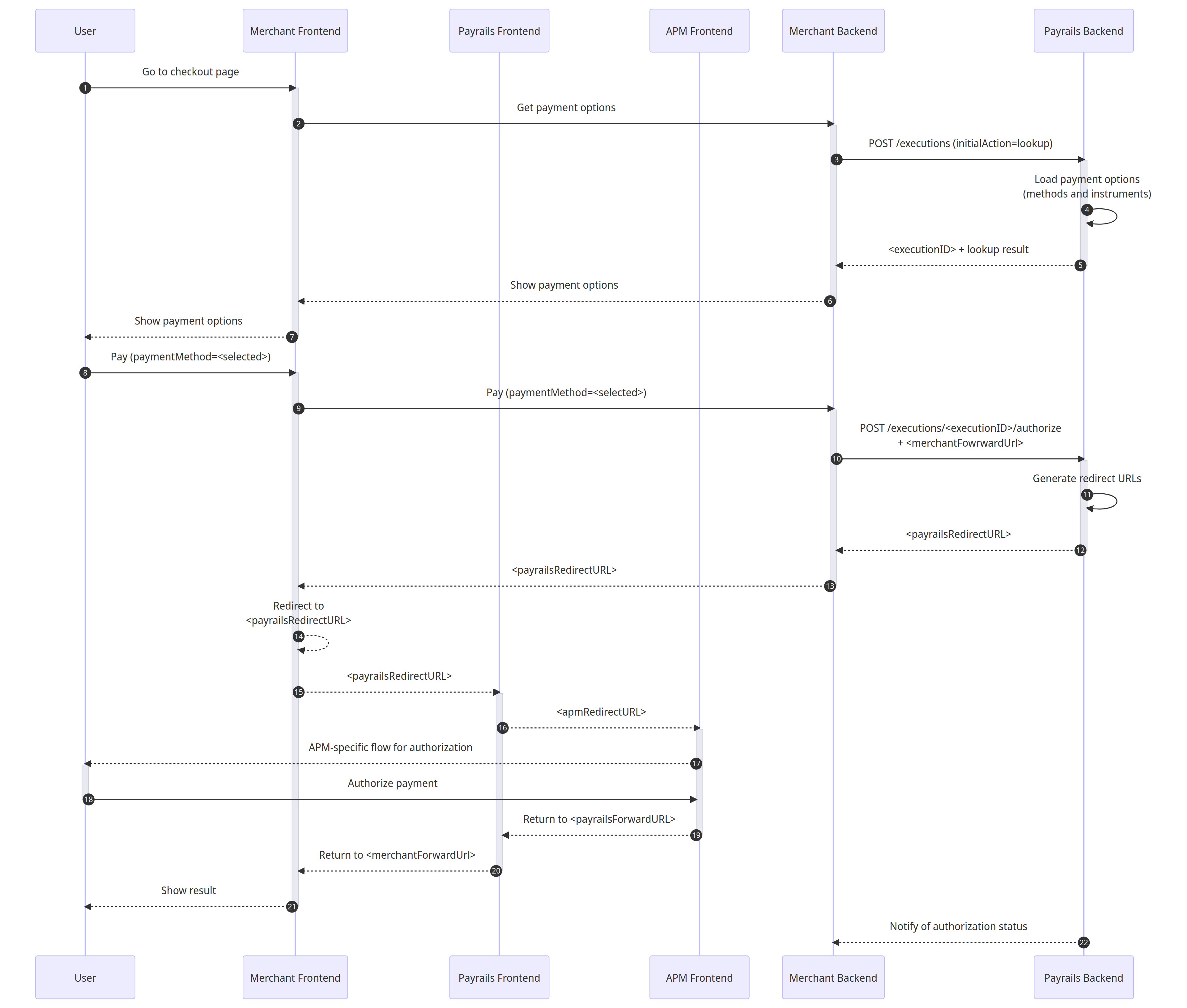
The APM redirect flow is similar to the 3-D Secure flow since both require a redirection.
Since the APM authorization happens in a WebView (mobile) or a webpage (web), the user journey will leave your domain. Therefore, you wanna make sure your authorization request specifies in the returnInfo
which return URL the user shall be redirected to after the APM authorization is complete.
After the authorization request is placed, you will be informed by a notification or long poll of the execution to fetch the status if a 3DS redirect was requested.
"actionRequired": "redirect",
"links": {
"redirect": "https://api.payrails.io/public/redirect/merchant/..."
}
After fetching the redirect URL, your client will open a WebView (mobile) or redirect the user to a webpage (web) where the challenge will take place.
When the challenge is resolved, Payrails will take your client back to the address you specified in the returnInfo
. It doesn't mean that your authorization was successful, but that it is in progress and Payrails will notify you about the success (or failure) later.
(Optional) Apple Pay and Google Pay
For Apple Pay and Google Pay, you will have to install their vendor SDK and fetch their payment token directly on the Client.
Then you pass the payment token with your Authorize a payment endpoint call.
Updated 7 months ago